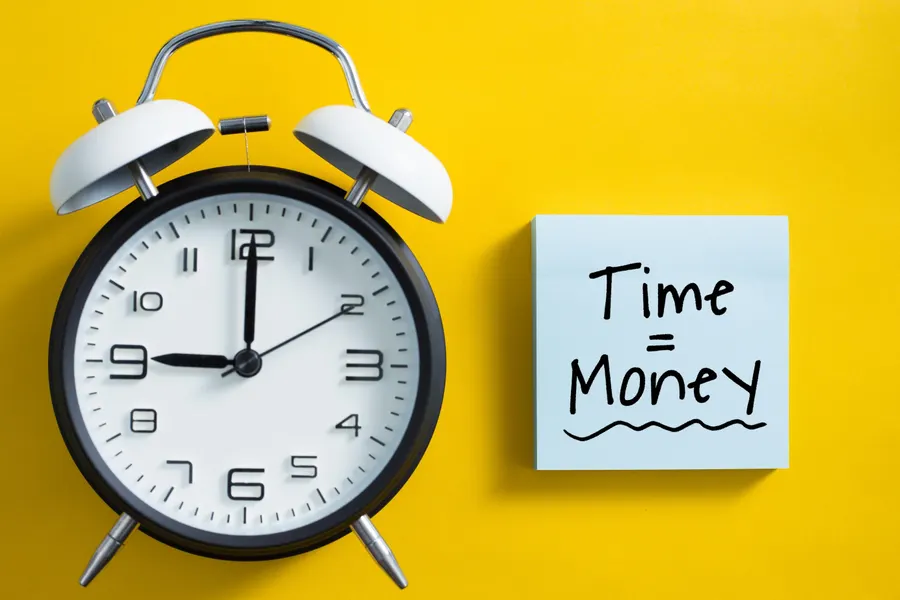
On the past posts we’ve introduced and explained some aspects about the evolution of computing services from on premises to cloud. The also quoted that time complexity is one of the keys to reduce costs in cloud computing.
Today we’re going to give you an example of how to implement a code improvement to reduce the running time of a function and reduce costs in cloud computing.
This example was written in Typescript, but the very important thing about it is the concept under the hood.
The problem:
Imagine a situation where you have a non ordered list of numbers and a target. The goal is to find out a pair of number on this list which is equal to the target value.

The output should be [0, 2] where it represents the indexes of the first two elements of the input list. Another thing is that there is just one answer.
One of the possible ways to solve this problem, maybe of the most intuitive could be create an index which will start in the begging of the input list and another one starting from the end of the list, going to middle of the list.
In each movement you should analise either the sum is equal or not to target value and decide increment the start index or decrement the end index. I think the code is very easy to understand.
The code below is the implementation of this algorithm.
function targetSum(nums: number[], target: number): number[] { let pStart: number = 0; let pEnd: number = nums.length - 1; const found: boolean = false; while (!found) { if (nums[pStart] + nums[pEnd] === target) { break; } if (nums[pEnd - 1] + nums[pStart] < nums[pStart + 1] + nums[pEnd]) { pEnd--; } else if (nums[pEnd - 1] + nums[pStart] === target) { pEnd--; break; } else if (nums[pStart + 1] + nums[pEnd] === target) { pStart++; break; } else { pStart++; } } const result = [pStart, pEnd]; return result; }
The code above run perfectly well, you’ll not receive any error from the compiler and so on, but… a capital BUT we’re focusing on reduce the running time, and this approach could be improved to reduce costs after all: TIME IS MONEY
The optimized solution
A best approach could be go in one direction from the begging to the end and calculate the differences between the current value and the target; after that store in a hashmap the current value of the input and its position. At each iteration, after calculate the difference, we look for the difference value on the hashmap. Take a look at the diagram below
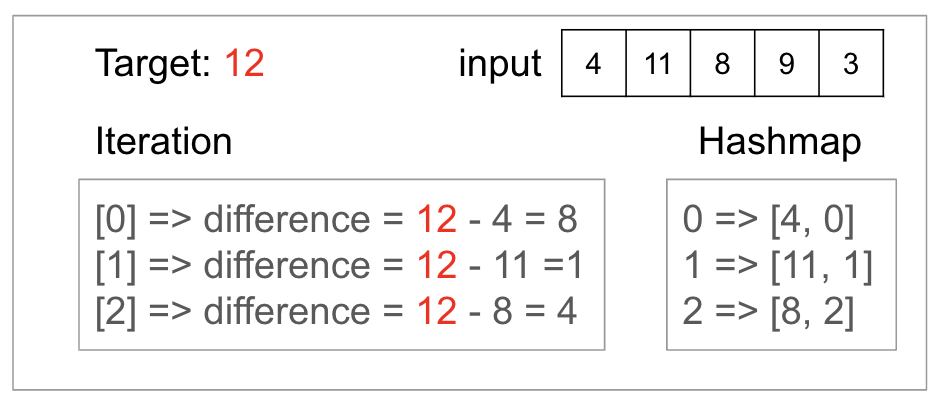
The implementation of the discussed code is very simple as you can see below:
function targetSumOpt(input: number[], target: number): number[] { const result = new Map(); for (let index = 0; index < input.length; index++) { const difference = target - input[index]; result.set(input[index], index); if (result.has(difference)) { return [result.get(difference), index]; } } return []; }
When we are talking about reduce cloud cost through code optimization there is no a cake recipe or silver bullet technique we must analise each case. There is a bunch of resources on computing’s bat belt and we’ll explain some of them in the next posts.
Hope you’re finding helpful this series.